설정(Configuration) 관련
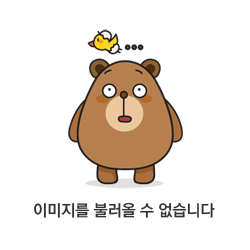
@Configuration
해당 클래스가 Spring Bean 구성 정보를 포함하고 있음을 나타냄
@Configuration을 클래스에 적용하고 @Bean을 method에 적용하여 @Autowired로 Bean 사용
@Configuration
public class AppConfig {
@Bean
public MyService myService() {
return new MyService();
}
@Bean
public MyRepository myRepository() {
return new MyRepository();
}
}
@Service
public class MyService {
private final MyRepository myRepository;
@Autowired
public MyService(MyRepository myRepository) {
this.myRepository = myRepository;
}
// 다른 메서드들
}
@Service
public class MyRepository {
// Repository 내용
}
@EnableAutoConfiguration
자동 설정을 활성화하여 클래스 패스 및 설정을 기반으로 자동으로 빈을 등록하고 구성
@Configuration
@EnableAutoConfiguration
public class MyConfiguration {
// 여기에 추가적인 설정을 할 수 있음
}
@Bean
개발자가 직접 제어가 불가능한 외부 라이브러리등을 Bean으로 만들려할 때 사용
@Configuration
public class AppConfig {
@Value("${my.feature.enabled}")
private boolean featureEnabled;
@Bean
public MyBean myBean() {
return new MyBean();
}
@Conditional(FeatureEnabledCondition.class)
@Bean
public FeatureEnabledBean featureEnabledBean() {
return new FeatureEnabledBean();
}
}
@Value
빈(Bean)의 범위(scope) 곧, 해당 빈 인스턴스의 생명주기를 정의
환경 프로퍼티(environment properties), 프로퍼티 파일(properties files), 시스템 프로퍼티(system properties), SpEL(스프링 표현 언어)에 있는 속성 값(property value)을 주입하는 데 사용
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Component
public class MyComponent {
@Value("${my.property}")
private String myProperty;
public void printProperty() {
System.out.println("my.property: " + myProperty);
}
}
my.property=Hello, Spring!
@Component
public class MyComponent {
public void printMessage(@Value("${my.message}") String message) {
System.out.println("my.message: " + message);
}
}
@PropertySource
빈(Bean)의 범위(scope) 곧, 해당 빈 인스턴스의 생명주기를 정의
외부 프로퍼티 파일을 Spring 애플리케이션 컨텍스트에 로드하고, 그 프로퍼티 값을 사용할 수 있도록 함
외부 환경 설정 파일을 읽고 애플리케이션에서 사용할 수 있는 속성을 정의
import org.springframework.context.annotation.PropertySource;
import org.springframework.context.annotation.Configuration;
@Configuration
@PropertySource("classpath:myapp.properties")
public class AppConfig {
// 여기에서 myapp.properties 파일에 정의된 속성 값을 사용할 수 있음
}
@Configuration
@PropertySources({
@PropertySource("classpath:app-defaults.properties"),
@PropertySource("classpath:app-overrides.properties")
})
public class AppConfig {
// 프로퍼티 파일들을 순서대로 로드하고 오버라이딩함
}
@Scope
빈(Bean)의 범위(scope) 곧, 해당 빈 인스턴스의 생명주기를 정의
Singleton, Prototype, Request, Session, Custom
@Component
@Scope("singleton")
public class MySingletonBean {
// ...
}
@Component
@Scope("prototype")
public class MyPrototypeBean {
// ...
}
@Component
@Scope("request")
public class MyRequestScopedBean {
// ...
}
@Component
@Scope("session")
public class MySessionScopedBean {
// ...
}
@Required
빈(bean) 설정 시에 반드시 필요한 프로퍼티(property)를 표시하는데 사용
해당 프로퍼티가 설정되지 않으면 빈을 초기화하는 동안 예외를 발생 (BeanInitializationException)
Spring 5.0 버전부터 deprecated되었으며, 대신 @Required 어노테이션을 사용하는 대신 @Autowired나 생성자 주입(Constructor Injection)을 사용하는 것이 권장
public class Employee {
private String name;
public String getName() {
return name;
}
@Required
public void setName(String name) {
this.name = name;
}
}
public class Employee {
private String name;
public Employee(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
@Qualifier
빈(bean) 주입 시에 어떤 빈을 사용할지 명시적으로 지정
동일한 타입의 빈이 여러 개 정의되어 있을 때 어떤 빈을 주입할지를 선택
public interface Animal {
void sound();
}
@Component
@Qualifier("cat")
public class Cat implements Animal {
public void sound() {
System.out.println("야옹");
}
}
@Component
@Qualifier("dog")
public class Dog implements Animal {
public void sound() {
System.out.println("멍멍");
}
}
@Service
public class AnimalService {
private final Animal cat;
private final Animal dog;
public AnimalService(@Qualifier("cat") Animal cat, @Qualifier("dog") Animal dog) {
this.cat = cat;
this.dog = dog;
}
public void makeSounds() {
cat.sound(); // "야옹" 출력
dog.sound(); // "멍멍" 출력
}
}
'learn > spring' 카테고리의 다른 글
어노테이션(Annotation) - Spring MVC 관련 (0) | 2023.09.26 |
---|---|
어노테이션 (Annotation) - AOP(Aspect-Oriented Programming) 관련 (0) | 2023.09.26 |
어노테이션(Annotation) - 의존성 주입(Dependency Injection) 관련 (0) | 2023.09.21 |
어노테이션(Annotation) - 컴포넌트 스캔(Component Scan) 관련 (0) | 2023.09.21 |
빈 (Bean) (2) | 2023.09.21 |